PHP Basics: What’s an Object?
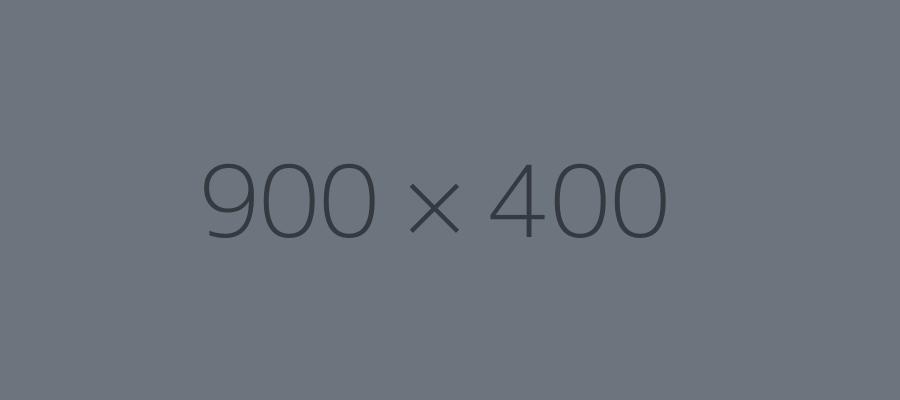
What is an object?
In PHP (and Joomla), an object is like a box that holds multiple values (called properties) and functions (called methods). An object is a suitcase full of useful info. You just have to open it and see what’s inside.
Example: The $article Object
When you load an article in Joomla, Joomla stores it in a variable like this:
$article = $this->item;
This $article is an object, and it contains all the info about that article:
-
Title
-
Intro text
-
Author
-
Publish dates
-
Category
-
And more!
How do we know what’s inside?
Use var_dump() or print_r() to look inside:
<?php
var_dump($article);
?>
That shows:
object(stdClass)#123 (15) {
["id"] => int(101)
["title"] => string(20) "Hello World Article"
["alias"] => string(11) "hello-world"
["introtext"] => string(250) "<p>Welcome to Joomla!</p>"
...
}
Accessing a property
Once you know what’s inside, you can grab individual values using the arrow syntax ->:
<?php
echo $article->title; // Shows the article title
echo $article->introtext; // Shows the intro text
?>
Common $article Properties
Property | Description |
---|---|
$article->title |
The article’s title |
$article->id |
The article's id number |
$article->introtext |
The intro/preview content |
$article->fulltext |
The full content (after "read more") |
$article->created_by |
The user ID who created it |
$article->hits |
Number of views (hit counter) |