PHP Basics: Understanding foreach Loops
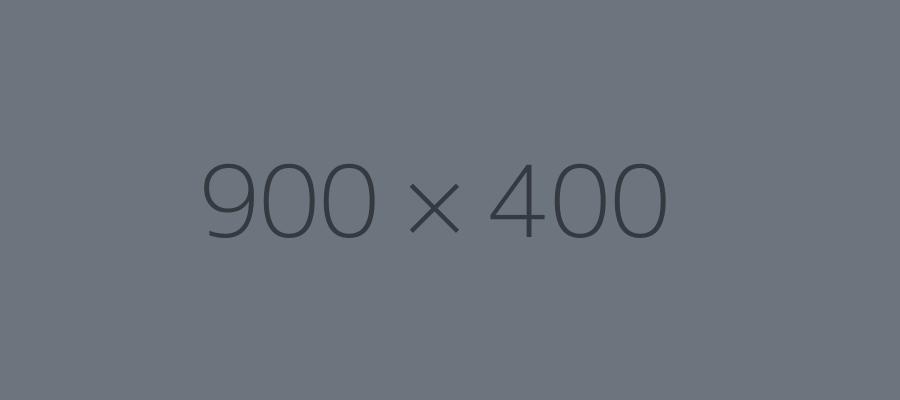
What is a foreach loop?
A foreach loop is a way to go through every item in an array, one at a time. Think of it like this: You have a tray of cookies (array). foreach lets you pick up each one, look at it, eat it, or do whatever you want—without knowing how many cookies there are.
<?php
foreach ($array as $item) {
// Do something with $item
}
?>
-
$array
is the list of things -
$item
is each individual thing in the list as the loop runs
Example 1: Loop through a simple array
<?php
$flavors = ['Milk', 'Dark', 'White'];
foreach ($flavors as $flavor) {
echo "I love $flavor chocolate!<br>";
}
?>
Output: I love Milk chocolate!
I love Dark chocolate!
I love White chocolate!
Example 2: Loop with both key and value
Sometimes you want to know the key (index or label), too:
<?php
$prices = ['Milk' => 3.99, 'Dark' => 4.49, 'White' => 4.29];
foreach ($prices as $type => $price) {
echo "$type Chocolate: \$$price<br>";
}
?>
Output: Milk Chocolate: $3.99
Dark Chocolate: $4.49
White Chocolate: $4.29
Example 3: Foreach in Joomla (articles)
<?php
foreach ($this->items as $article) {
echo "<h3>" . $article->title . "</h3>";
echo "<p>" . $article->introtext . "</p>";
}
?>
This would display all the articles loaded by the Joomla component or module.
Common Mistakes
-
❌ Forgetting the
as
keyword -
❌ Using the wrong variable name inside the loop
-
❌ Assuming
$item
is always a string—it could be an array or object
If you don’t know how many things are in a list, or if you want to loop over them all, foreach is your best friend.