PHP Basics: Understanding if and else
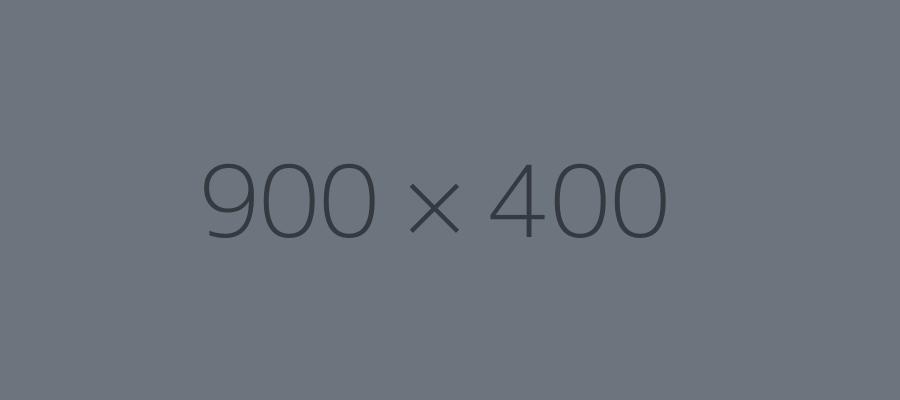
What is an if statement?
if lets you make decisions in your code.
Basic Structure
<?php
if (condition) {
// do this if true
} else {
// do this if false
}
?>
Example
<?php
$age = 20;
if ($age >= 18) {
echo "You’re old enough to vote!";
} else {
echo "Sorry, come back when you're older.";
}
?>
Output: You’re old enough to vote!
Using else if
Need more than just yes or no? Use elseif to check extra conditions:
<?php
$score = 85;
if ($score >= 90) {
echo "You got an A!";
} elseif ($score >= 80) {
echo "You got a B!";
} else {
echo "Keep trying!";
}
?>
Output: You got a B!
Comparison Operators You’ll Use
Symbol | Meaning | Example ($x = 10 ) |
---|---|---|
== |
Equal to | $x == 10 ✅ |
!= |
Not equal to | $x != 5 ✅ |
> |
Greater than | $x > 5 ✅ |
< |
Less than | $x < 15 ✅ |
>= |
Greater than or equal to | $x >= 10 ✅ |
<= |
Less than or equal to | $x <= 20 ✅ |
Common Mistakes
-
❌ Using
=
instead of==
=
sets a value,==
compares it!
✅ Correct:if ($x == 5)
-
❌ Missing curly braces
{}
Always use them for clarity, even for one-liners!
Real-World Example (with HTML)
<?php
$isLoggedIn = false;
if ($isLoggedIn) {
echo "<p>Welcome back!</p>";
} else {
echo "<p>Please log in.</p>";
}
?>
Output:
Please log in.
Practice if/else with different values and conditions. It’s one of the most important building blocks in PHP!