PHP Basics: Understanding Echo
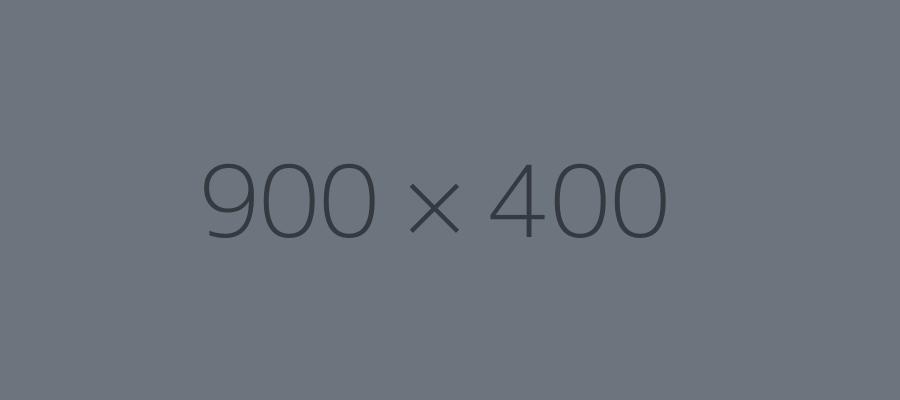
What is echo in PHP?:
echo is a command in PHP that outputs text to the screen. It’s one of the most common and easiest ways to display something—like a sentence, number, or HTML—on a web page. Think of it like saying, “Hey PHP, show this!”
Basic Syntax:
<?php
echo "Hello, world!";
?>
Output: Hello, world!
- The text inside the quotes is what you’ll see on the page.
- Every PHP statement ends with a semicolon (;).
Double vs. Single Quotes:
You can use either:
<?php echo "This works!";?>
<?php echo 'So does this!';?>
Tip: If you want to include variables (like someone’s name), double quotes are better. Example:
<?php
$name = "Alex";
echo "Hello, $name!"; // Outputs: Hello, Alex!
?>
Printing HTML:
You can also output HTML tags:
<?php echo "<h1>Welcome to my site</h1>";?>
That shows up as a big headline in your browser.
Multiple echos or Combined Text:
<?php
echo "Hello, ";
echo "world!";
?>
or combine:
<?php
echo "Hello, " . "world!";
?>
The . is the concatenation operator (it joins pieces of text together).
Common Mistakes:
Forgetting the semicolon:
<?php echo "Hi"?>
Real-World Example:
<?php
$flavor = "dark chocolate";
echo "<p>I love $flavor!</p>";
?>
Output in browser:
<p>I love dark chocolate!</p>